TypeScript vs JavaScript: Understanding the Differences and Choosing the Right Language for Your Development Projects
Introduction: Why Understanding TypeScript and JavaScript Matters
JavaScript and TypeScript are two of the most important languages in the modern web development landscape. The more complex your projects become, the more you’ll appreciate which one is stronger and which one is weaker so that you make the proper decision in building your applications.
JavaScript is a flexible and dynamic language capable of running within browsers and on servers. It’s an excellent choice when speed is key for rapid development, and its “massive” ecosystem of libraries and frameworks doesn’t hurt either. Still, in an application of a size approaching significance, the lack of a strict type system really hurts- at least when tracing through errors.
TypeScript is JavaScript extended with static types. It allows for catching errors during the development process, rather than at runtime. That makes it especially useful for large projects where the maintainability of the code and long-term scalability will be crucial.
By the end of this blog, you will have learned what is TypeScript vs JavaScript brings to the table and how to pick one against the other based on what your project needs.
2. History and Evolution
JavaScript:
JavaScript was created by Brendan Eich in 1995 when he was working at Netscape. Its original purpose was to add interactivity to web pages using simple scripts running in the browser. It was for a long time considered a less elaborate language and, at times could even be belittled as a toy. However, with the web becoming more dynamic, JavaScript has evolved into being the primary language for building rich, interactive sites.
Node.js was officially launched back in 2009, expanding the capabilities of JavaScript and transforming it into a full-stack language. According to W3Techs, today, JavaScript is used by around 97% of all websites-that is, a fully first-choice web programming language.
TypeScript:
In 2012, Microsoft developed TypeScript to deal with the increasing complexity of applications built in JavaScript. JavaScript applications started becoming more applicable, and codebases were becoming really big issues; JavaScript was dynamically typed, which made it hard to maintain because of its size. This is where TypeScript brought static typing along with other features, such as interfaces and classes, to assist the developers in writing more solid and maintainable code.
It is built as a superset for JavaScript, i.e., it inherits all the features of JavaScript while adding additional syntax to it towards static typing. Since it compiles down to plain JavaScript, TypeScript can be incrementally introduced into existing JavaScript projects.
3. Syntax and Language Features
JavaScript Features:
Dynamic Typing:
In JavaScript, the type of a variable is dynamically typed, so its type can be changed at runtime as well. A variable that had been defined to be a numeric type would later on be assigned to be of string type, and so on. This sometimes brings flexibility but also unifies hard-to-spot bugs:
javascript
Copy code
let value = “Hello!”;
value = 100; // No error, despite the type having changed.
This feature of dynamism makes it easier to code at a higher speed but in turn, increases the possibilities of runtime errors.
Prototype-based Object Orientation:
Java Script follows prototype-based object-oriented programming. It allows any JavaScript object to have a prototype; therefore, objects can inherit properties and methods from other objects. As such, it is slightly tougher for developers trained in class-based languages like Java or C++ to get a full understanding of the concept.
ES6+ Features Modern JavaScript boasts powerful features that have been introduced in ECMAScript 6 and later. These include the likes of arrow functions, destructuring, template literals, and classes, making JavaScript more intuitive and easier to write. For example, the syntax of the arrow function makes it easier to write an anonymous function like this:
javascript
Copy
const greet = () => console.log(“Hello, World!”);
Static Typing with Type Annotations:
One of the most notable features of TypeScript is its static typing. You explicitly declare the types of variables, functions, and objects so that at compile time, long before the code runs, the TypeScript compiler can catch type-related errors. This saves countless hours spent in debugging.
Other Advanced Features:
TypeScript, like many others, has the following advanced features, including interfaces, enums, and generics. These features mean that the shape of the object should be defined with strict contracts so that the code is more predictable and maintainable. For example, in TypeScript interface vs type will ensure that an object follows the pattern below:
typescript
Copy code
interface Person {
name: string;
age: number;
}
let person: Person = { name: “John”, age: 30 }; // Valid
ES6+ Support:
TypeScript supports all the latest JavaScript features, starting with ES6, so developers can always use the best JavaScript has to offer, combined with the benefits of static type checking.
4. Tooling and Development Experience
JavaScript:
Node.js and npm:
The JavaScript environment depends extensively on Node.js for server-side scripting and npm (Node Package Manager) for managing third-party libraries and dependencies. Today, more than 1.3 million packages are available in npm, which makes JavaScript developers enjoy the unprecedented dispersion of tools, libraries, and frameworks that accelerate development.
IDE Support:
One good number of IDEs is used for JavaScript, such as VS Code, WebStorm, Sublime Text, and Atom. These environments provide essential features, like debugging, syntax highlighting, and code completion, to make work simpler with JavaScript.
TypeScript:
TypeScript Compiler (tic):
As the name suggests, browsers do not natively support it, so there needs to be a compilation step with the help of the TypeScript compiler, etc. There’s some added overhead to this, but on the other hand, a compiler can catch bugs earlier at compile-time.
Enhanced IDE Support:
Modern IDEs, like Visual Studio Code, have great support for TypeScript, along with attributes such as intelligent code completion, real-time error checking, and auto refactoring, which make working with TypeScript highly efficient and less error-prone.
Create React App with TypeScript:
When it comes to React applications, the create-react-app tool comes with TypeScript templates by default, so it is easier to add TypeScript to new React projects right away in create react app typescript:
bash
Copy code
npx create-react-app my-app –template typescript
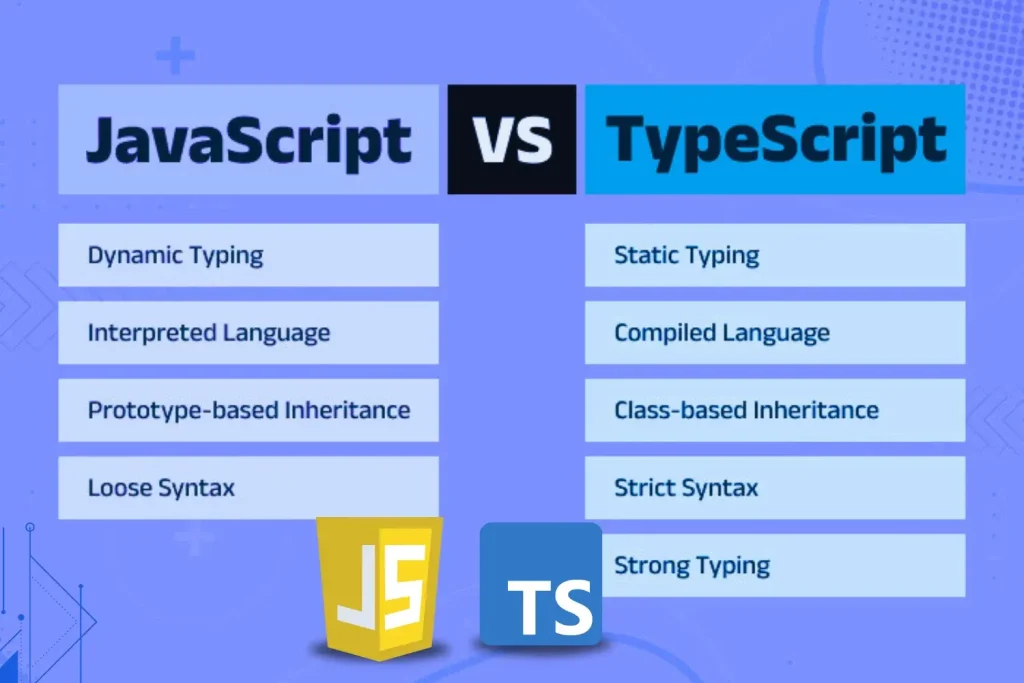
5. Type System and Benefits
JavaScript’s Dynamic Typing:
Benefits:
JavaScript’s dynamic typing is excellent for fast prototyping and flexibility. That allows you to write code quickly without paying too much attention to giving the types so it is very good for the small project or where you just need to iterate over something quick.
Challenges:
The downside to dynamic typing is that errors may only be present during runtime. For example, something as minor as a typo or an unintended data type can be problematic to debug. It may lead to problems, which means an otherwise fairly large codebase in JavaScript might be more difficult to maintain down the line.
TypeScript’s Static Typing:
Advantages:
Static typing of TypeScript supports defining the types of variables, function parameters, and object properties. That makes the code more predictable and decreases the
Number of possible runtime errors:
Typescript
Copy
function greet(name: string): void {
console.log(`Hello, ${name}!`);
}
Greet (123); // Error: Argument of type ‘number’ is not assignable to parameter of type ‘string’.
Early Error Detection:
It catches many different forms of errors at compile time before the code is ever run, and that helps you avoid a lot of bugs due to mismatched data types; this way, your code is more reliable and maintainable, particularly in larger applications.
Static typing makes the code much clearer, especially when working in teams because everyone now clearly understands what a particular variable or function is supposed to represent. In general, it makes refactoring safer and easier, which allows for truly scalable applications.
6. Compatibility and Interoperability
JavaScript Compatibility:
Browser Support:
In addition, JavaScript runs on every major browser without needing extra tools. Whether you are building a website or mobile application or even designing a desktop application using Electron, JavaScript works without needing extra tools. It also seamlessly interacts with HTML and CSS, making it the natural choice for front-end development.
Framework and Language Integration :
JavaScript plays nice with nearly any framework (React, Vue, Angular), as well as
nearly all other languages (HTML, CSS, and even back-end languages, like Python or
PHP) even the people think what is the difference between java and python but in
the end developers can build completely functional full-stack applications using
JavaScript only.
TS Compatibility:
Compiles to JavaScript:
It compiles down to plain JavaScript, hence completely compatible with all browsers. This means that it can be used in any kind of JavaScript environment: TypeScript code compiled can run anywhere JavaScript code can run.
Incremental Adoption:
Another strength of TypeScript is that it may be adopted gradually in the existing JavaScript projects. You might begin to introduce the type annotations into a file one by one without rewriting your entire codebase. In this way, it becomes easier to introduce the new system into an ongoing project.
7. Performance and Efficiency
JavaScript Performance:
Native Performance:
Browsers directly interpret JavaScript, and modern JavaScript engines such as V8 in Chrome are incredibly fast and efficient. A lot of evolution in JavaScript performance takes place and continues to improve with the introduction of new features with optimisations for faster execution.
Perfect Suitable for Smaller Projects:
Since JavaScript is dynamically typed, there is no compilation step needed that can make development go faster with quicker iteration through the process of coding. This makes JavaScript appropriate for smaller projects, as the performance overhead is not a major concern.
TypeScript Performance:
Minimal Runtime Overhead:
Since TypeScript compiles down to JavaScript, the runtime performance is the same as JavaScript. The benefits of static typing in Typescript come at development time rather than at runtime; thus, you get the advantage of more maintainable and error-free code without sacrificing production performance.
Light Compilation Overhead:
The only potential inefficiency is additional development time to compile TypeScript into JavaScript. Fortunately, most modern build tools, including Webpack and Babel, make this process seamless.
8. Community and Ecosystem
JavaScript Ecosystem:
Huge Community:
JavaScript has been in use for decades, and it has one of the biggest communities worldwide. There are thousands of tutorials, resources, open-source libraries, and frameworks available for JavaScript, so it becomes easier to find a solution for your problems and get help whenever needed. The JavaScript community is also super active and sees some of the most fascinating contributions toward open-source projects, which starts up innovation in this language.
Vast Ecosystem:
With over 1.3 million packages in npm, JavaScript is home to a really massive library and tool ecosystem that reaches almost every part of web and application development. Not only does it contain huge UI libraries like React, but even server-side frameworks like Express are there.
TypeScript Ecosystem:
A vibrant and rapidly growing community
Whereas the TypeScript community is much smaller in size compared to the JavaScript community, it is growing at a pretty rapid pace. As reported by the 2022 Stack Overflow Developer Survey, TypeScript is among the most beloved languages by developers. More and more open-source projects, frameworks, and libraries are being written in TypeScript or offering TypeScript support.
Enterprise Adoption:
It has been widely adopted in enterprises and large development teams due to its focus on code quality and maintainability. Large-scale applications at companies like Microsoft, Google, and Airbnb use TypeScript.
9. Use Cases and Adoption
When to Use JavaScript:
Small to Medium-Sized Projects:
If you have something on the smaller side in terms of project size, if you need rapid prototyping, you should be looking at JavaScript. The flexibility of dynamic typing is good for rapidly building and iterating without the hassle of declaring types or compiling code.
Client-Side Scripting:
JavaScript is the principal language for client-side scripting, which means it is really their first choice for interactive user interfaces, changing DOM content, and responding to events, such as clicks or form submissions.
Full-Stack Development:
With frameworks like Node.js, JavaScript can be used both for the front-end and back-end of an application, hence in a unified stack.
When to Use TypeScript:
Large-Scale Applications:
TypeScript does fantastic in large applications. In such large applications, maintainability, consistency, and scalability will be very important. The type system will allow new developers to understand the codebase easily, and refactoring becomes much safer.
Enterprise Development:
The emphasis of TypeScript on type safety often makes enterprises choose it over others because it reduces the number of bugs developed in long-term projects and, more importantly, increases productivity among developers.
Long-Term Projects:
TypeScript should be your choice if you find your project to change with time. Static typing in TypeScript helps new features get integrated without breaking the existing functionality.
10. Learning Curve and Adoption
JavaScript Learning Curve:
Easy to Begin:
JavaScript is one of the easiest languages to begin with. In fact, it is very commonly taught as a first programming language because of its lenient syntax and flexibility. Even beginners can quickly build interactivity into web pages using basic JavaScript string conation skills like:
Steep Advanced Learning Curve:
Although it is relatively easy to learn JavaScript, a lot of hard work is required to master it. Some complicated concepts of closure, asynchronous programming, and an event loop seem daunting for a newcomer. Flexibility creates a problem as a code base grows, and developers need to learn how to enable javascript really in large projects.
TypeScript Learning Curve:
Initial Higher Learning Curve
This sometimes means an overwhelming syntax and feature set based on the background of a developer who may be learning static typing for the first time. The concepts of interfaces, generics, and type annotations need more studying.
Well, Worth the Investment:
Although having a higher learning curve compared to JavaScript, catching errors earlier and making the system more maintainable pay for the effort spent learning this knowledge by developers doing large or complex projects.
11. Future Trends and Updates
JavaScript Future:
Continuing with the Evolution:
The evolution of JavaScript never appears to have an end. New standards in ECMAScript are continually coming out. Features such as optional chaining, bullish coalescing, and top-level await really improved usability and readability of the language. It’s also being optimised; in fact, modern engines like V8 and SpiderMonkey push the bar further to what was supposed to be impossible in terms of performance.
TypeScript Future:
Growth of the Future is Bright:
No, TypeScript seems not to slow down anytime soon. Each new version includes new, very useful features, among which type inference bettering, improved compiler performance, and much more refined static analysis stand out. Much as its future is tied up with the evolution of JavaScript, TypeScript is going to enjoy new and improved features of JavaScript, more advanced static typing features.
12. Decision Making and Recommendations
When to Use JavaScript:
Use JavaScript if you are creating:
Small projects in which flexibility and fast prototyping are more important than rigid-type safety.
Applications that are client-side, for example, when using a framework like React, Vue, or Angular.
Projects in which you must rapidly iterate based on feedback and deploy quickly without too much focus on forward scalability.
When to Use TypeScript:
Use TypeScript if you are:
For large-scale or enterprise projects where long-term maintainability is a primary requirement, you build.
For projects where you need multiple developers, where the priority is to ensure that coding standards are consistent and that bugs get reduced considerably, you build.
For complex applications where a scalable, maintainable codebase is essential, such as an e-commerce platform, a data-heavy dashboard, or some enterprise-level software you build.
13. Conclusion
Finally, the choice is between JavaScript and TypeScript, and both are good and bad. JavaScript is adaptive and dynamic and great for small projects or when you need rapid, flexible development. With regard to more significant projects or long-term projects where reducing bugs and increasing maintainability is critical, TypeScript comes with more structure and safety on the table.
Knowing the difference between TypeScript and JavaScript will thus help you choose the right tool to implement for a project. Both languages are incredibly valuable tools in a modern developer’s arsenal, all ready to be the right tool for the job, whether you are just starting or creating the most complex, intricate enterprise-level applications.
Frequently Asked Questions
TypeScript is usually compiled to JavaScript; thus, performance is the same, with differences taking place at development time, not runtime.
JavaScript is older, dating back from 1995, whereas TypeScript was first released in 2012.
TypeScript provides static typing and better tooling, which makes it easier to write high-quality code, maintain it well, and have productive developers.
With the TypeScript safety types, the React components will be endowed with type safety, making it easier to catch mistakes during development than in pure JavaScript.
TypeScript is a better fit for both front-end and back-end development. It offers strong typing, which makes code much more reliable on every application.